Smart Contract for Token Distribution: Code and Explanation
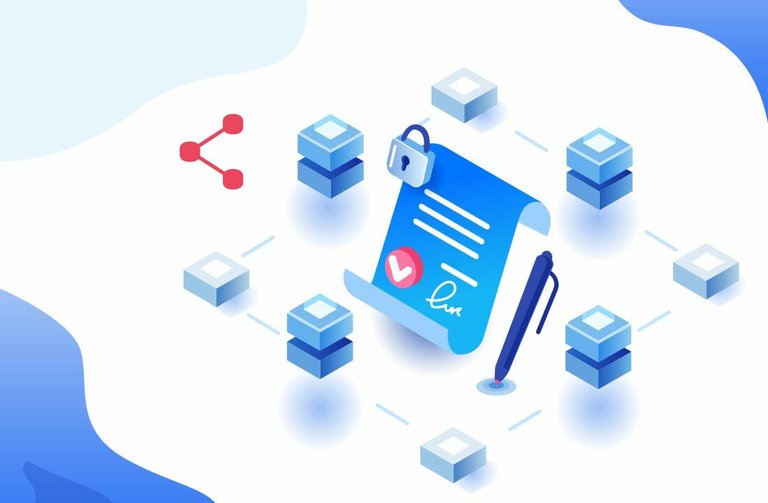
Here is a sample smart contract for token distribution on the Ethereum blockchain using the Solidity programming language. Then I explain each line.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract TokenDistribution {
address public owner;
mapping(address => uint256) public balances;
event TokensDistributed(address indexed to, uint256 amount);
constructor() {
owner = msg.sender;
}
modifier onlyOwner() {
require(msg.sender == owner, "Only the owner can execute this function");
_;
}
function distributeTokens(address[] memory recipients, uint256[] memory amounts) public onlyOwner {
require(recipients.length == amounts.length, "Recipients and amounts arrays must have the same length");
for (uint256 i = 0; i < recipients.length; i++) {
balances[recipients[i]] += amounts[i];
emit TokensDistributed(recipients[i], amounts[i]);
}
}
function getBalance(address account) public view returns (uint256) {
return balances[account];
}
}
// SPDX-License-Identifier: MIT
- This line specifies the license under which the contract is released. Here, it is the MIT license.
pragma solidity ^0.8.0;
- This line specifies the version of the Solidity compiler to be used. It ensures compatibility with Solidity version 0.8.0 or higher.
contract TokenDistribution {
- This line declares a new contract named
TokenDistribution
.
- This line declares a new contract named
address public owner;
- This line declares a public variable
owner
of typeaddress
to store the address of the contract owner.
- This line declares a public variable
mapping(address => uint256) public balances;
- This line declares a public mapping named
balances
that maps addresses to their token balances.
- This line declares a public mapping named
event TokensDistributed(address indexed to, uint256 amount);
- This line declares an event named
TokensDistributed
that logs the distribution of tokens.
- This line declares an event named
constructor() {
- This line declares a constructor function that is executed once when the contract is deployed.
owner = msg.sender;
- This line sets the
owner
variable to the address that deployed the contract.
- This line sets the
modifier onlyOwner() {
- This line declares a modifier named
onlyOwner
to restrict access to certain functions.
- This line declares a modifier named
require(msg.sender == owner, "Only the owner can execute this function");
- This line checks if the caller of the function is the owner. If not, it throws an error.
_
- This line is a placeholder for the function body that uses the
onlyOwner
modifier.
- This line is a placeholder for the function body that uses the
function distributeTokens(address[] memory recipients, uint256[] memory amounts) public onlyOwner {
- This line declares a public function named
distributeTokens
that takes two arrays as parameters:recipients
andamounts
.
- This line declares a public function named
require(recipients.length == amounts.length, "Recipients and amounts arrays must have the same length");
- This line checks if the lengths of the
recipients
andamounts
arrays are equal. If not, it throws an error.
- This line checks if the lengths of the
for (uint256 i = 0; i < recipients.length; i++) {
- This line starts a for loop to iterate over the
recipients
array.
- This line starts a for loop to iterate over the
balances[recipients[i]] += amounts[i];
- This line updates the balance of each recipient by adding the corresponding amount.
emit TokensDistributed(recipients[i], amounts[i]);
- This line emits the
TokensDistributed
event for each recipient.
- This line emits the
function getBalance(address account) public view returns (uint256) {
- This line declares a public view function named
getBalance
that returns the token balance of a given account.
- This line declares a public view function named
return balances[account];
- This line returns the balance of the specified account.