Start Python Game Programming By albro
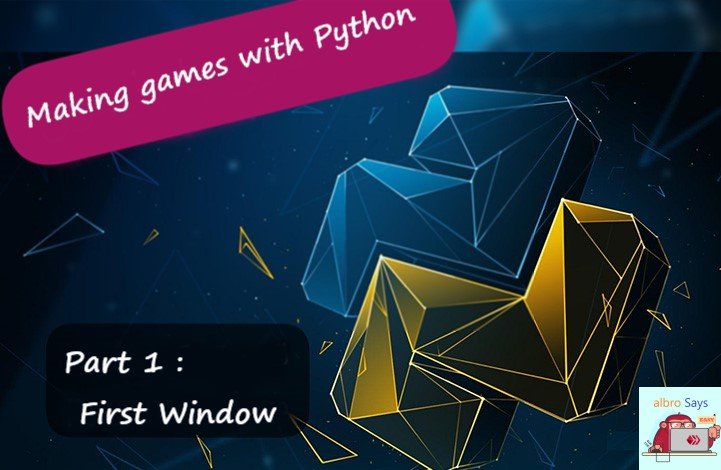
This section is for those who are familiar with Python programming and are interested in expanding their knowledge in game development. We will start with the basics of making games with pygame, which is a Python library, and then implement our knowledge in the form of several projects.
What is the game?
The most important entertainment of all of us in childhood were games. Games have helped us to develop our physical and mental skills. Games are the best simulation of the real world in which we can practice living. They even teach us how to treat friends and enemies. They're the best, most interesting and at the same time the most effective way to teach skills.
When we play our favorite game, we enter another world; A world that may be more real for us than our own world at that moment. We put ourselves in the place of their characters, we fight, love, fail and win in their place. In this world, all the impossible things become possible, and maybe that's why it's hard to give up on them.
Games have a long history and are seen in all cultures and nations and represent the culture and thoughts of each nation. Games have special rules. These rules make them different from other games. For example, the rules of the chess game are different from the OXO game, and their shape is also different. Games are played for various purposes, such as entertainment, challenge, education or earning, etc. Games are a combination of art, creativity, literature, psychology and even math and physics knowledge. They rely on mental, physical or luck skills; Like games played with dice.
Three things can be considered for each game:
- The players
- Rules of the game
- Game environment
We know that games are generally of two categories: computer games and real world games. All computer games are directly and indirectly made by coding, and all code is written and executed in the same programming language. In this post, I'm going to understand how to make a game with the attractive and widely used Python language.
Making games with Python
There are many ways to make games with Python. Since we all like the shortest path more, I want to review pygame.
Pygame is an open source Python library for building multimedia applications. pygame is a hybrid library of C, Python, Native and OpenGL. The cross-platform feature of SDL and pygame allows us to write games and multimedia programs for any platform.
Other libraries for creating games with Python
As I said, pygame isn't the only way to make games with Python. You can use the following libraries for this.
- PyKyra : PyKyra is one of the fastest game development frameworks for Python, built on both SDL and the Kyra engine. This framework also supports MPEG, MP3, Ogg Vorbis, Wav, images and more.
- Pyglet: Pyglet is an open source multimedia library for Python. pyglet is a powerful Python library for developing games and other multimedia applications. It can be used on Windows, Mac OS X and Linux. It supports windowing, event management, OpenGL graphics, loading images and videos, and playing audio and music. Pyglet runs on Python 3.5+.
- PyOpenGL: PyOpenGL is a graphics library that is supported by operating systems such as Windows, Linux and MacOS. PyOpenGL is compatible with a large number of external Python GUI libraries such as PyGame, PyQt, Raw XLib.
- Kivy: Kivy is an open-source and cross-platform Python library for rapid application development. Kivy runs on Linux, Windows, OS X, Android, iOS and Raspberry Pi and can support most protocols and devices including WM_Touch, WM_Pen, Mac OS X Trackpad and Magic Mouse, Mtdev, Linux Kernel HID and Use TUIO.
- Panda3D: Panda3D is an open source and completely free engine for 3D games, visualization, simulation, testing and more. Panda3D includes command line tools for processing and optimization. Panda3D supports many popular third-party libraries such as Bullet, Assimp, OpenAL and FMOD, among others.
What is a package manager?
A package manager or package-management system is a set of software tools that continuously install, update, configure, and remove computer programs for a computer operating system. A package manager deals with packages, software distribution, and data in archive files.
Packages have metadata, such as the name of the software, description of its work, version of the software, its provider, checksum (which is usually an encrypted hash function) and a list of dependencies necessary for the correct execution of the software.
After installing the package, the metadata is stored in a local database of the package. Package managers usually maintain a database of software dependencies and version information to avoid software incompatibilities and missing prerequisites. They work closely with software repositories, binary managers, repositories and app stores.
Package managers are designed to install and update packages automatically. In the image below, you can see some different package managers:
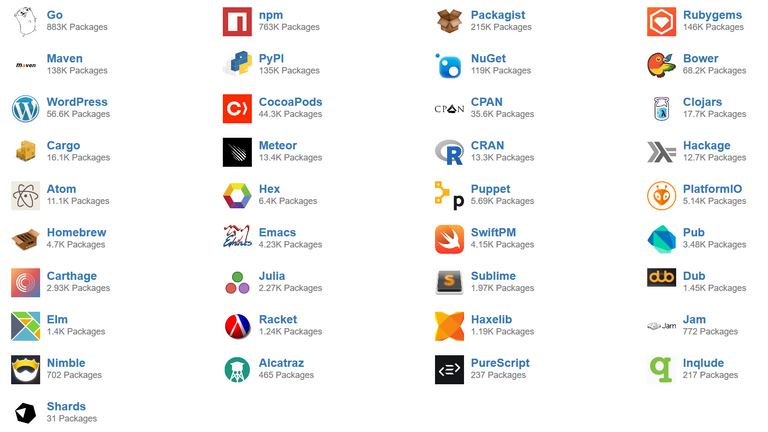
What is pip?
pip is a package manager for python, like npm for javascript and composer for php, etc. External packages can be installed and downloaded using pip.
Installing pygame on Windows
As you know, pygame is an external python library and isn't installed in the system along with python itself. To install pygame, we need to install it with the python package manager, i.e. pip. For this, we open the terminal or cmd again and write the command to install the library, i.e. pip install pygame
, and press Enter. To install pygame, it doesn't matter which drive we are on:

If the installation of pygame is done correctly, you should see the image above.
How to Create A Simple Window with Pygame?
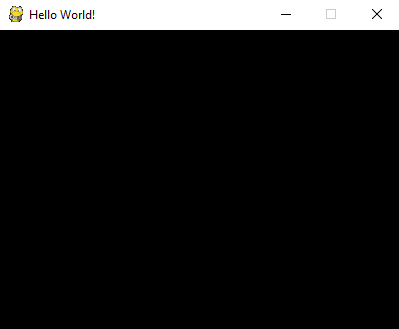
The first program I write with pygame is the small program above, which is called "Hello World!". To start, I open a code editor. I create a new file named helloworld.py
and write the following codes in it and then save it. You can use any text editor you want. Let's take a look at the code.
import pygame, sys
from pygame.locals import *
pygame.init()
DISPLAYSURF = pygame.display.set_mode((200, 300))
pygame.display.set_caption('Hello World!')
while True: # main game loop
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
pygame.display.update()
Importing the libraries and constants of the locals
module
To use an external library in Python, we must import it into the program. For example, if we want to use the math
module, we must import it into the program with the statement import math
. So, to use pygame
and sys
, we must import them. as below:
import pygame,sys
The above line is present in every pygame program. This line is a simple import
that imports the pygame
and sys
modules into the program so that our program can use the functions in them. All pygame functions that deal with graphics, sound, etc. are in the pygame
module. All you need to know about the sys
module is that it contains variables and functions related to the Python interpreter.
In the next line, we enter pygame.locals
into the program:
from pygame.locals import *
The reason we use pygame.locals
is that we need the constants in it. The pygame.locals
module contains 280 constants. Placing this statement at the beginning of the program will import them all into the program. Like QUIT
which I've used in my program.
Initialize pygame
With the following line, we actually want to tell Python that we intend to use pygame. For this, we need to initialize pygame. If we don't do this, pygame won't work. The pygame.init
code should always come after the pygame
module and before any other functions. You don't need to know what this function does, just know that in order for pygame functions to work properly, this function must be called before them. So we have:
pygame.init()
Determine the size of the window and its name
The next line is a call to the display.set_mode
function, which returns an object called pygame.Surface
for the window. In fact, set_mode
initializes a Screen
object to display the window. We send a double tuple of two integers to the function: (400,300)
. This tuple specifies the height and width of the set_mode
function to create the window. (400,300)
creates a window 400
pixels width and 300
pixels height. So the first number is for the width and the second number is for the height, and both must be integer or int
.
Don't use these two numbers alone in the set_mode
function and make sure you put them in a tuple or an array. The correct way to call this function is as follows:
DISPLAYSURF=pygame.display.set_mode((400,300))
Using the above code as pygame.display.set_mode(400, 300)
causes an error as follows:

The above error says that the argument passed to the function must be a double tuple or an array with two integer values. So always remember to put the window size in a tuple or array and then use it in set_mode
. The object returned from set_mode
is stored in a variable called DISPLAYSURF
.
In the following line, we have determined the name of the window with the pygame.display.set_caption
function. The name of the window appears above it. This function takes a string as a parameter. The string 'Hello World!'
is passed to this function:
pygame.display.set_caption('Hello World!')

Loop and game state
while True: # main game loop
for event in pygame.event.get():
The above line is a while
loop whose condition is True
. This loop never stops unless its condition is False
. The only way to exit the loop is to execute a break
or sys.exit()
. All the games in these posts have this while
loop. We call this loop the main loop of the game. Its job is to run the game until the window is closed. Inside the main loop, there is another loop, which we call the event management loop. This loop is the most important part of a reactive program like games. The event handler loop does three things:
- Handles events such as clicking the mouse or pressing a button on the click screen and...
- Updates or edits the game state.
- Shows the game status on the screen.
Take a good look at the picture below to better understand the above writings:
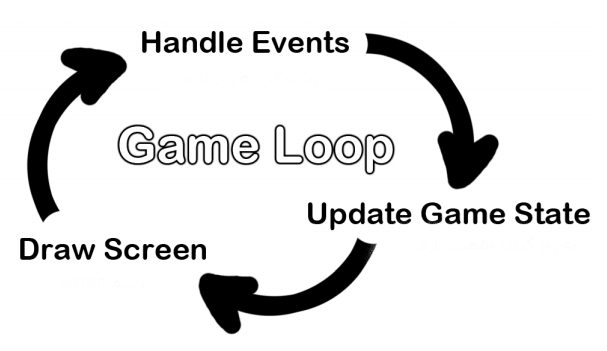
What is the game state?
If you've ever played a game that allows you to save stages and not have to start from the first stage every time you play, I'd say you have a little familiarity with game mode.
Game state is a simple way to refer to all the variables in a game. In many games, the state of the game contains the values of variables such as the number of lives, the position of the players and the position of the enemies, which are usually shown at the top or bottom of the screen with a special shape. Whenever an event occurs, such as a player being damaged (which reduces one of his lives), the enemy moving, or ... that changes the value of game variables, we say that the game state has changed.
The game state remains at the point where you saved it. In most games, pausing the game will not change the state of the game. Since game state is usually updated in response to events (such as mouse clicks or keyboard presses) or the passage of time, the game loop must check every second for each new event that has occurred. pygame does this checking with the pygame.event.get
function. The event loop has code that updates the game state based on the generated event. This is called event handling or event management. So the name of the event loop comes from what it does.
pygame.event.Event
objects (checking events and managing them)
Every time the user performs several actions together, such as pressing the keyboard or moving the mouse over the application window, a pygame.event.Event
object is created by the pygame
library to record these events. (The Event
object is in the event
module, and the event
module itself is in the pygame
module.) We can find out which events have occurred by calling the pygame.event.get()
function. This function returns an array of pygame.event.Event
objects.
The members of this array will have two states :(read the following two sentences several times)
- In the first case, its members are equal to the events that have occurred since the last call to the
pygame.event.get
function. - If
pygame.event.get
has never been called, the events that have occurred since the beginning of the program will form the members of the array.
The for
loop loops in the event
array returned by the pygame.event.get
function, that is, it repeats a series of statements several times. In each iteration of the for
loop, the event
variable specifies an event in the event
array. The event
array returns the events in the order they occurred. (Note that event
variable and event array
are different.)
If the user clicks the mouse and then presses a key on the keyboard, the event object
will be the first member of the array for the mouse click and the second member for the keyboard press. that's mean:
event[0] = Event object for mouse click
event[1] = Event object for the key pressed
pygame.event.get
returns an empty array
if no event has occurred.
QUIT
event and pygame.quit
function
if event.type==QUIT:
pygame.quit()
sys.exit()
Event
objects have an attribute called type
that specifies the type of event. pygame has a constant for each of these types in the pygame.locals
module. The above line of code checks whether the event type is equal to the constant value QUIT
or not. If we have a quit event, then the pygame.quit
and sys.exit
functions are called. The pygame.quit
function is kind of the opposite of the pygame.init
function. This function executes the code that disables the pygame library.
The pygame.quit
function must always precede sys.exit
. Here we don't have any conditions for the rest of the events like mouse movement and... so if you click on the window or press one of the buttons, nothing happens.
update
function (updating game status)
pygame.display.update()
The last line calls the pygame.display.update
function. This function displays the object returned by pygame.display.set_mode
(remember that we stored this object in the DISPLAYSURF
variable). Since the DISPLAYSURF
object does not change in the game, every time the pygame.display.update
function is called, the same black image is displayed again. After the last line is executed, the while
loop repeats its task of displaying the black window. To close the window, we must click on the X
button from the corner of the window.
Finally, I must say that all programs written with pygame have three general and fixed parts:
- Importing libraries and initializing pygame with
pygame.init
- Definition of variables and constants
- The main loop of the game
These three sections are shown in the following image:
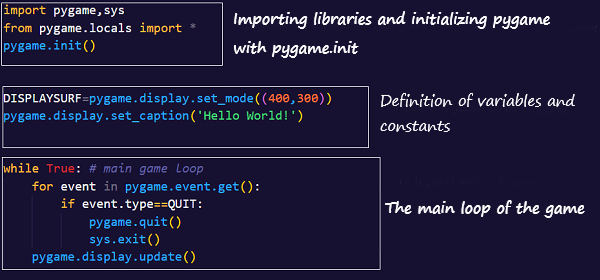
https://leofinance.io/threads/albro/re-leothreads-2wedqskfd
The rewards earned on this comment will go directly to the people ( albro ) sharing the post on LeoThreads,LikeTu,dBuzz.
Congratulations @albro! You have completed the following achievement on the Hive blockchain And have been rewarded with New badge(s)
Your next target is to reach 300 upvotes.
You can view your badges on your board and compare yourself to others in the Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
To support your work, I also upvoted your post!
Congratulations!
✅ Good job. Your post has been appreciated and has received support from CHESS BROTHERS ♔ 💪
♟ We invite you to use our hashtag #chessbrothers and learn more about us.
♟♟ You can also reach us on our Discord server and promote your posts there.
♟♟♟ Consider joining our curation trail so we work as a team and you get rewards automatically.
♞♟ Check out our @chessbrotherspro account to learn about the curation process carried out daily by our team.
🥇 If you want to earn profits with your HP delegation and support our project, we invite you to join the Master Investor plan. Here you can learn how to do it.
Kindly
The CHESS BROTHERS team
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.